concepts::InOutParameters Class Reference
Holds parameters in hashes. More...
#include <inputOutput.hh>
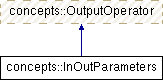
Public Member Functions | |
void | addArrayBool (const char *array, const bool newArray=false) |
Creates an emtpy array for bools if necessary. More... | |
void | addArrayBool (const char *array, const int number, const int value) |
Adds a bool to an array. More... | |
void | addArrayComplex (const char *array, const bool newArray=false) |
Creates an empty array for complex doubles if necessary. More... | |
void | addArrayComplex (const char *array, const int number, const std::complex< double > value) |
Adds a complex double to an array. More... | |
void | addArrayDouble (const char *array, const bool newArray=false) |
Creates an empty array for doubles if necessary. More... | |
void | addArrayDouble (const char *array, const int number, const double value) |
Adds a double to an array. More... | |
void | addArrayInt (const char *array, const bool newArray=false) |
Creates an empty array for ints if necessary More... | |
void | addArrayInt (const char *array, const int number, const int value) |
Adds an int to an array. More... | |
void | addArrayString (const char *array, const bool newArray=false) |
Creates an empty array for strings if necessary. More... | |
void | addArrayString (const char *array, const int number, const char *value) |
Adds a string to an array. More... | |
void | addBool (const char *name, const int value) |
Adds a bool to the hash of bools. More... | |
void | addComplex (const char *name, const std::complex< double > value) |
Adds a complex double to the hash of doubles. More... | |
void | addDouble (const char *name, const double value) |
Adds a double to the hash of doubles. More... | |
void | addInt (const char *name, const int value) |
Adds an int to the hash of ints. More... | |
void | addMatrixDouble (const char *array, const bool newMatrix=false) |
Creates an empty matrix for doubles if necessary. More... | |
void | addMatrixDouble (const char *matrix, const int i, const int j, const double value) |
Adds a double to an matrix. More... | |
void | addMatrixInt (const char *array, const bool newMatrix=false) |
Creates an empty matrix for integer if necessary. More... | |
void | addMatrixInt (const char *matrix, const int i, const int j, const int value) |
Adds a integer to an matrix. More... | |
void | addString (const char *name, const char *value) |
Adds a string to the hash of strings. More... | |
void | append (const InOutParameters &inout, bool arrayAppend=true) |
Appends inout . More... | |
void | clear () |
clears the object More... | |
bool | getArrayBool (const char *array, const int number) const |
Returns a bool from an array. More... | |
std::complex< double > | getArrayComplex (const char *array, const int number) const |
Returns a complex double from an array. More... | |
double | getArrayDouble (const char *array, const int number) const |
Returns a double from an array. More... | |
int | getArrayInt (const char *array, const int number) const |
Returns a int from an array. More... | |
std::string | getArrayString (const char *array, const int number) const |
Returns a string from an array. More... | |
bool | getBool (const char *name) const |
Returns a bool from the hash of bools. More... | |
bool | getBool (const char *name, const bool value) const |
Returns a bool from the hash of bools. More... | |
std::complex< double > | getComplex (const char *name) const |
Returns a complex double from the hash of doubles. More... | |
double | getDouble (const char *name) const |
Returns a double from the hash of doubles. More... | |
double | getDouble (const char *name, const double value) const |
Returns a double from the hash of doubles. More... | |
int | getInt (const char *name, const int value=INT_MAX) const |
Returns an int from the hash of ints. More... | |
const std::map< int, bool > & | getMapBool (const char *array) const |
Returns a reference to the requested map. More... | |
const std::map< int, std::complex< double > > & | getMapComplex (const char *array) const |
Returns a reference to the requested map. More... | |
const std::map< int, double > & | getMapDouble (const char *array) const |
Returns a reference to the requested map. More... | |
const std::map< int, int > & | getMapInt (const char *array) const |
Returns a reference to the requested map. More... | |
const std::map< int, std::string > & | getMapString (const char *array) const |
Returns a reference to the requested map. More... | |
double | getMatrixDouble (const char *array, const int i, const int j) const |
Returns a double from an matrix. More... | |
int | getMatrixInt (const char *array, const int i, const int j) const |
Returns a integer from an matrix. More... | |
std::string | getString (const char *name, const char *value=0) const |
Returns a string from the hash of strings. More... | |
InOutParameters () | |
Constructor. More... | |
InOutParameters (const InOutParameters &i) | |
Copy constructor. More... | |
void | storeMatlab (const char *filename, const std::string description="") const |
Output as matlab function, which gives a object back. More... | |
std::ostream & | storeMatlab (std::ostream &os, const char *name, const std::string description="") const |
Output as matlab function, which gives a object back. More... | |
virtual | ~InOutParameters () |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
Private Member Functions | |
template<class F > | |
void | add_ (std::map< const char *, F, ltstr > &map, const char *name, const F value) |
Adds a entrance value of type F to field name . More... | |
template<class F , class G , class H > | |
void | addArray_ (std::map< const char *, std::map< F, G, H > *, ltstr > &map, const char *array, const bool newArray=false) |
template<class F , class G , class H > | |
void | addArray_ (std::map< const char *, std::map< F, G, H > *, ltstr > &map, const char *array, const F number, const G value) |
template<class F > | |
void | clear_ (std::map< const char *, F, ltstr > &map) |
clears one mapping More... | |
template<class F , class G > | |
void | clear_ (std::map< const char *, std::map< G, F > *, ltstr > &map) |
template<class F > | |
F | get_ (const std::map< const char *, F, ltstr > &map, const char *name) const |
template<class F , class G , class H > | |
G | getArray_ (const std::map< const char *, std::map< F, G, H > *, ltstr > &map, const char *array, const F number) const |
template<class F > | |
const std::map< int, F > & | getMap_ (const std::map< const char *, std::map< int, F > *, ltstr > &map, const char *array) const |
template<class F > | |
void | mapArrayOutputMatlab_ (std::ostream &os, const std::map< const char *, std::map< int, F > *, ltstr > &map, const std::string brackets="[]") const |
Matlab output of one mapping of an array. More... | |
template<class F > | |
void | mapMatrixOutputMatlab_ (std::ostream &os, const std::map< const char *, std::map< MultiIndex< 2 >, F, ltidx > *, ltstr > &map, const std::string brackets="[]") const |
Matlab output of one mapping of an matrix. More... | |
template<class F > | |
void | mapOutputMatlab_ (std::ostream &os, const std::map< const char *, F, ltstr > &map) const |
Matlab output of one mapping. More... | |
Private Attributes | |
std::map< const char *, bool, ltstr > | bool_ |
Hash of bools. More... | |
std::map< const char *, std::map< int, bool > *, ltstr > | boolArrays_ |
Hash of arrays of bools. More... | |
std::map< const char *, std::complex< double >, ltstr > | complex_ |
Hash of complex doubles. More... | |
std::map< const char *, std::map< int, std::complex< double > > *, ltstr > | complexArrays_ |
Hash of arrays of complex. More... | |
std::map< const char *, double, ltstr > | double_ |
Hash of doubles. More... | |
std::map< const char *, std::map< int, double > *, ltstr > | doubleArrays_ |
Hash of arrays of doubles. More... | |
std::map< const char *, std::map< MultiIndex< 2 >, double, ltidx > *, ltstr > | doubleMatrices_ |
Hash of matrices of doubles. More... | |
std::map< const char *, int, ltstr > | int_ |
Hash of ints. More... | |
std::map< const char *, std::map< int, int > *, ltstr > | intArrays_ |
Hash of arrays of ints. More... | |
std::map< const char *, std::map< MultiIndex< 2 >, int, ltidx > *, ltstr > | intMatrices_ |
Hash of matrices of integer. More... | |
std::map< const char *, std::string, ltstr > | string_ |
Hash of strings. More... | |
std::map< const char *, std::map< int, std::string > *, ltstr > | stringArrays_ |
Hash of arrays of strings. More... | |
Detailed Description
Holds parameters in hashes.
This class has four hashes to hold integer, double, bool and string parameters and four hashes to hold arrays of integers, doubles, bools and strings. It does therefore store (name, value) pairs in the hashes and (name, number, value) triples in the arrays.
The main usage of this class is by inputParser which reads an input file and enters the parameters into such an object.
If a parameter already exists and is added a second time, the value is simply overwritten. The same is true for the entries in the arrays. If an array is created a second time, nothing happens.
The output operator writes the contents of the whole class to the output stream in the format of the input file. The output is not in the order of the input file.
- See also
- inputParser
- Examples
- cig_load_input_data.cc, elasticity2D_tutorial.cc, hpFEM2d.cc, hpFEM3d-EV.cc, inputoutput.cc, linearDG1d.cc, and linearFEM1d.cc.
Definition at line 75 of file inputOutput.hh.
Constructor & Destructor Documentation
◆ InOutParameters() [1/2]
|
inline |
Constructor.
Definition at line 78 of file inputOutput.hh.
◆ InOutParameters() [2/2]
concepts::InOutParameters::InOutParameters | ( | const InOutParameters & | i | ) |
Copy constructor.
◆ ~InOutParameters()
|
virtual |
Member Function Documentation
◆ add_()
|
private |
Adds a entrance value
of type F
to field name
.
◆ addArray_() [1/2]
|
private |
◆ addArray_() [2/2]
|
private |
◆ addArrayBool() [1/2]
void concepts::InOutParameters::addArrayBool | ( | const char * | array, |
const bool | newArray = false |
||
) |
Creates an emtpy array for bools if necessary.
If newArray
is true, the array entries will be deleted
◆ addArrayBool() [2/2]
void concepts::InOutParameters::addArrayBool | ( | const char * | array, |
const int | number, | ||
const int | value | ||
) |
Adds a bool to an array.
- Parameters
-
array Name of the array number Number of the entry value Value of the entry
- Precondition
- The array must exist
◆ addArrayComplex() [1/2]
void concepts::InOutParameters::addArrayComplex | ( | const char * | array, |
const bool | newArray = false |
||
) |
Creates an empty array for complex doubles if necessary.
If newArray
is true, the array entries will be deleted
◆ addArrayComplex() [2/2]
void concepts::InOutParameters::addArrayComplex | ( | const char * | array, |
const int | number, | ||
const std::complex< double > | value | ||
) |
Adds a complex double to an array.
- Parameters
-
array Name of the array number Number of the entry value Value of the entry
- Precondition
- The array must exist
◆ addArrayDouble() [1/2]
void concepts::InOutParameters::addArrayDouble | ( | const char * | array, |
const bool | newArray = false |
||
) |
Creates an empty array for doubles if necessary.
If newArray
is true, the array entries will be deleted
- Examples
- hpFEM2d.cc, hpFEM3d-EV.cc, inputoutput.cc, linearDG1d.cc, and linearFEM1d.cc.
◆ addArrayDouble() [2/2]
void concepts::InOutParameters::addArrayDouble | ( | const char * | array, |
const int | number, | ||
const double | value | ||
) |
Adds a double to an array.
- Parameters
-
array Name of the array number Number of the entry value Value of the entry
- Precondition
- The array must exist
◆ addArrayInt() [1/2]
void concepts::InOutParameters::addArrayInt | ( | const char * | array, |
const bool | newArray = false |
||
) |
Creates an empty array for ints if necessary
If newArray
is true, the array entries will be deleted
- Examples
- hpFEM2d.cc, and hpFEM3d-EV.cc.
◆ addArrayInt() [2/2]
void concepts::InOutParameters::addArrayInt | ( | const char * | array, |
const int | number, | ||
const int | value | ||
) |
Adds an int to an array.
- Parameters
-
array Name of the array number Number of the entry value Value of the entry
- Precondition
- The array must exist
◆ addArrayString() [1/2]
void concepts::InOutParameters::addArrayString | ( | const char * | array, |
const bool | newArray = false |
||
) |
Creates an empty array for strings if necessary.
If newArray
is true, the array entries will be deleted
◆ addArrayString() [2/2]
void concepts::InOutParameters::addArrayString | ( | const char * | array, |
const int | number, | ||
const char * | value | ||
) |
Adds a string to an array.
- Parameters
-
array Name of the array number Number of the entry value Value of the entry
- Precondition
- The array must exist
◆ addBool()
void concepts::InOutParameters::addBool | ( | const char * | name, |
const int | value | ||
) |
Adds a bool to the hash of bools.
- Examples
- elasticity2D_tutorial.cc, hpFEM2d.cc, and inputoutput.cc.
◆ addComplex()
void concepts::InOutParameters::addComplex | ( | const char * | name, |
const std::complex< double > | value | ||
) |
Adds a complex double to the hash of doubles.
◆ addDouble()
void concepts::InOutParameters::addDouble | ( | const char * | name, |
const double | value | ||
) |
Adds a double to the hash of doubles.
- Examples
- hpFEM2d.cc, hpFEM3d-EV.cc, and linearFEM1d.cc.
◆ addInt()
void concepts::InOutParameters::addInt | ( | const char * | name, |
const int | value | ||
) |
Adds an int to the hash of ints.
- Examples
- elasticity2D_tutorial.cc, hpFEM2d.cc, hpFEM3d-EV.cc, inputoutput.cc, linearDG1d.cc, and linearFEM1d.cc.
◆ addMatrixDouble() [1/2]
void concepts::InOutParameters::addMatrixDouble | ( | const char * | array, |
const bool | newMatrix = false |
||
) |
Creates an empty matrix for doubles if necessary.
If newMatrix
is true, the array entries will be deleted
◆ addMatrixDouble() [2/2]
void concepts::InOutParameters::addMatrixDouble | ( | const char * | matrix, |
const int | i, | ||
const int | j, | ||
const double | value | ||
) |
Adds a double to an matrix.
- Parameters
-
array Name of the matrix i Row index of the entry in the matrix j Column index of the entry in the matrix value Value of the entry
- Precondition
- The matrix must exist
◆ addMatrixInt() [1/2]
void concepts::InOutParameters::addMatrixInt | ( | const char * | array, |
const bool | newMatrix = false |
||
) |
Creates an empty matrix for integer if necessary.
If newMatrix
is true, the array entries will be deleted
◆ addMatrixInt() [2/2]
void concepts::InOutParameters::addMatrixInt | ( | const char * | matrix, |
const int | i, | ||
const int | j, | ||
const int | value | ||
) |
Adds a integer to an matrix.
- Parameters
-
array Name of the matrix i Row index of the entry in the matrix j Column index of the entry in the matrix value Value of the entry
- Precondition
- The matrix must exist
◆ addString()
void concepts::InOutParameters::addString | ( | const char * | name, |
const char * | value | ||
) |
Adds a string to the hash of strings.
- Examples
- elasticity2D_tutorial.cc, hpFEM2d.cc, hpFEM3d-EV.cc, and inputoutput.cc.
◆ append()
void concepts::InOutParameters::append | ( | const InOutParameters & | inout, |
bool | arrayAppend = true |
||
) |
Appends inout
.
- Parameters
-
arrayAppend true: append array values, false: overwrite arrays
◆ clear()
void concepts::InOutParameters::clear | ( | ) |
clears the object
◆ clear_() [1/2]
|
private |
clears one mapping
◆ clear_() [2/2]
|
private |
◆ get_()
|
private |
◆ getArray_()
|
private |
◆ getArrayBool()
bool concepts::InOutParameters::getArrayBool | ( | const char * | array, |
const int | number | ||
) | const |
Returns a bool from an array.
- Parameters
-
array Name of the array number Number of the entry in the array
◆ getArrayComplex()
std::complex<double> concepts::InOutParameters::getArrayComplex | ( | const char * | array, |
const int | number | ||
) | const |
Returns a complex double from an array.
- Parameters
-
array Name of the array number Number of the entry in the array
◆ getArrayDouble()
double concepts::InOutParameters::getArrayDouble | ( | const char * | array, |
const int | number | ||
) | const |
Returns a double from an array.
- Parameters
-
array Name of the array number Number of the entry in the array
- Examples
- hpFEM2d.cc, and hpFEM3d-EV.cc.
◆ getArrayInt()
int concepts::InOutParameters::getArrayInt | ( | const char * | array, |
const int | number | ||
) | const |
Returns a int from an array.
- Parameters
-
array Name of the array number Number of the entry in the array
- Examples
- hpFEM2d.cc.
◆ getArrayString()
std::string concepts::InOutParameters::getArrayString | ( | const char * | array, |
const int | number | ||
) | const |
Returns a string from an array.
- Parameters
-
array Name of the array number Number of the entry in the array
- Examples
- hpFEM2d.cc.
◆ getBool() [1/2]
bool concepts::InOutParameters::getBool | ( | const char * | name | ) | const |
Returns a bool from the hash of bools.
- Examples
- cig_load_input_data.cc, and hpFEM2d.cc.
◆ getBool() [2/2]
bool concepts::InOutParameters::getBool | ( | const char * | name, |
const bool | value | ||
) | const |
Returns a bool from the hash of bools.
If name
does not exist take value
.
◆ getComplex()
std::complex<double> concepts::InOutParameters::getComplex | ( | const char * | name | ) | const |
Returns a complex double from the hash of doubles.
◆ getDouble() [1/2]
double concepts::InOutParameters::getDouble | ( | const char * | name | ) | const |
Returns a double from the hash of doubles.
- Examples
- cig_load_input_data.cc, elasticity2D_tutorial.cc, hpFEM2d.cc, hpFEM3d-EV.cc, and linearFEM1d.cc.
◆ getDouble() [2/2]
double concepts::InOutParameters::getDouble | ( | const char * | name, |
const double | value | ||
) | const |
Returns a double from the hash of doubles.
If name
does not exist take value
.
◆ getInt()
int concepts::InOutParameters::getInt | ( | const char * | name, |
const int | value = INT_MAX |
||
) | const |
Returns an int from the hash of ints.
If name
does not exist take value
.
◆ getMap_()
|
private |
◆ getMapBool()
const std::map<int, bool>& concepts::InOutParameters::getMapBool | ( | const char * | array | ) | const |
Returns a reference to the requested map.
- Exceptions
-
MissingParameter
◆ getMapComplex()
const std::map<int, std::complex<double> >& concepts::InOutParameters::getMapComplex | ( | const char * | array | ) | const |
Returns a reference to the requested map.
- Exceptions
-
MissingParameter
◆ getMapDouble()
const std::map<int, double>& concepts::InOutParameters::getMapDouble | ( | const char * | array | ) | const |
Returns a reference to the requested map.
- Exceptions
-
MissingParameter
◆ getMapInt()
const std::map<int, int>& concepts::InOutParameters::getMapInt | ( | const char * | array | ) | const |
Returns a reference to the requested map.
- Exceptions
-
MissingParameter
◆ getMapString()
const std::map<int, std::string>& concepts::InOutParameters::getMapString | ( | const char * | array | ) | const |
Returns a reference to the requested map.
- Exceptions
-
MissingParameter
◆ getMatrixDouble()
double concepts::InOutParameters::getMatrixDouble | ( | const char * | array, |
const int | i, | ||
const int | j | ||
) | const |
Returns a double from an matrix.
- Parameters
-
array Name of the matrix i Row index of the entry in the matrix j Column index of the entry in the matrix
◆ getMatrixInt()
int concepts::InOutParameters::getMatrixInt | ( | const char * | array, |
const int | i, | ||
const int | j | ||
) | const |
Returns a integer from an matrix.
- Parameters
-
array Name of the matrix i Row index of the entry in the matrix j Column index of the entry in the matrix
◆ getString()
std::string concepts::InOutParameters::getString | ( | const char * | name, |
const char * | value = 0 |
||
) | const |
Returns a string from the hash of strings.
If string name
does not exist take value
.
- Examples
- cig_load_input_data.cc, elasticity2D_tutorial.cc, hpFEM2d.cc, and hpFEM3d-EV.cc.
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Reimplemented from concepts::OutputOperator.
◆ mapArrayOutputMatlab_()
|
private |
Matlab output of one mapping of an array.
◆ mapMatrixOutputMatlab_()
|
private |
Matlab output of one mapping of an matrix.
◆ mapOutputMatlab_()
|
private |
Matlab output of one mapping.
◆ storeMatlab() [1/2]
void concepts::InOutParameters::storeMatlab | ( | const char * | filename, |
const std::string | description = "" |
||
) | const |
Output as matlab function, which gives a object back.
- Parameters
-
filename name of the output file, ending .m is added
◆ storeMatlab() [2/2]
std::ostream& concepts::InOutParameters::storeMatlab | ( | std::ostream & | os, |
const char * | name, | ||
const std::string | description = "" |
||
) | const |
Output as matlab function, which gives a object back.
- Parameters
-
os output stream name name of the function
Member Data Documentation
◆ bool_
|
private |
Hash of bools.
Definition at line 333 of file inputOutput.hh.
◆ boolArrays_
|
private |
Hash of arrays of bools.
Definition at line 345 of file inputOutput.hh.
◆ complex_
|
private |
Hash of complex doubles.
Definition at line 327 of file inputOutput.hh.
◆ complexArrays_
|
private |
Hash of arrays of complex.
Definition at line 339 of file inputOutput.hh.
◆ double_
|
private |
Hash of doubles.
Definition at line 325 of file inputOutput.hh.
◆ doubleArrays_
|
private |
Hash of arrays of doubles.
Definition at line 336 of file inputOutput.hh.
◆ doubleMatrices_
|
private |
Hash of matrices of doubles.
Definition at line 349 of file inputOutput.hh.
◆ int_
|
private |
Hash of ints.
Definition at line 331 of file inputOutput.hh.
◆ intArrays_
|
private |
Hash of arrays of ints.
Definition at line 343 of file inputOutput.hh.
◆ intMatrices_
|
private |
Hash of matrices of integer.
Definition at line 352 of file inputOutput.hh.
◆ string_
|
private |
Hash of strings.
Definition at line 329 of file inputOutput.hh.
◆ stringArrays_
|
private |
Hash of arrays of strings.
Definition at line 341 of file inputOutput.hh.
The documentation for this class was generated from the following file:
- toolbox/inputOutput.hh