timestepping::TimeVector Class Reference
Class implementing time dependent vectors. More...
#include <vectors.hh>
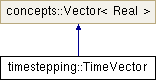
Public Member Functions | |
virtual Function< Real > & | add (const Function< Real > &fnc, Real sc) |
Vector< Real > & | add (const Vector< H > &fnc) |
Adds a vector of possible different length and type. More... | |
Vector< Real > & | add (const Vector< H > &fnc, Real sc, uint offset=0) |
Adds a vector of possible different length and type with an offset. More... | |
Vector< Real > & | apply (Real fnc(const Real &)) |
Application operator to each component, e.g. More... | |
Vector< Real > & | assemble (const Space< G > &spc, const LinearForm< Real, G > &lf) |
Assembles the vector w.r.t. linear form lf and space spc . More... | |
Real * | data () const |
Real | l1 () const |
l1 norm More... | |
Real | l2 () const |
l2 norm More... | |
Real | l2_2 () const |
l2 norm squared More... | |
Real | max () const |
Maximum of the absolute values in the vector, ie. ![]() | |
uint | n () const |
Elements in the vector. More... | |
operator Real * () const | |
Conversion operator. More... | |
virtual Real & | operator() (uint i) |
virtual Real | operator() (uint i) const |
Real | operator* (const Vector< Real > &fnc) const |
Inner product (v = this , w = \fnc) More... | |
Vector< Real > | operator* (Real c) const |
virtual Function< Real > & | operator*= (Real sc) |
Scaling operator. More... | |
Vector< Real > | operator+ (const Function< Real > &fnc) const |
Vector< Real > | operator+ (Real c) const |
virtual Function< Real > & | operator+= (const Function< Real > &fnc) |
Addition operator. More... | |
virtual Function< Real > & | operator+= (Real c) |
Vector< Real > | operator- (const Function< Real > &fnc) const |
Vector< Real > | operator- (Real c) const |
virtual Function< Real > & | operator-= (const Function< Real > &fnc) |
Subtraction operator. More... | |
virtual Function< Real > & | operator-= (Real c) |
Vector< Real > | operator/ (Real c) const |
virtual Function< Real > & | operator/= (Real sc) |
virtual void | resize (uint n) |
Sets a new size, previous data might be lost More... | |
void | reverse () |
uint | size () const |
void | storeMatlab (const char *filename, const char *name=0, bool append=false) const |
Stores the vector in a Matlab sparse matrix. More... | |
Real | time () const |
Returns the current time_ . More... | |
void | time (Real time) |
Sets the current time_ . More... | |
TimeVector (const concepts::Space< Real > &spc) | |
Constructor. More... | |
TimeVector (const concepts::Space< Real > &spc, TimeLinearForm &tlf) | |
Constructor. More... | |
TimeVector (const concepts::Vector< Real > &vec) | |
Copy Constructor. More... | |
TimeVector (const concepts::Vector< Real > &vec, const concepts::Formula< Real > &frm, const Real time=0) | |
Constructor for a constant vector times a formula in time. More... | |
const Vector< Real > & | write (const std::string &fname) const |
Writes the vector to a file. More... | |
~TimeVector () | |
Protected Member Functions | |
void | fillEntries_ (const Space< G > &spc, const LinearForm< Real, G > &lf) |
virtual std::ostream & | info (std::ostream &os) const |
Protected Attributes | |
Array< Real > | data_ |
Vector data. More... | |
Private Member Functions | |
void | set_ (const concepts::Vector< Real > &vec) |
Sets the vector to vec . More... | |
void | updateEntries_ () |
Evaluates tlf_ at time_ . More... | |
Private Attributes | |
const concepts::Formula< Real > * | frm_ |
Formula in time to multiply with template vector. More... | |
const concepts::Space< Real > * | spc_ |
Space. More... | |
const concepts::Vector< Real > * | template_ |
Template vector. More... | |
Real | time_ |
Time, is not necessarily correlated to the values of the vector. More... | |
TimeLinearForm * | tlf_ |
Linear form. More... | |
Real * | v_ |
Pointer to the vector data. More... | |
Static Private Attributes | |
static uint | storeMatlabCounter_ |
Counts number of Matlab outputs (used to uniquely name the vectors) More... | |
Detailed Description
Class implementing time dependent vectors.
In order to get the vector at time t, call the time function with argument t.
Definition at line 53 of file vectors.hh.
Constructor & Destructor Documentation
◆ TimeVector() [1/4]
|
inline |
Constructor.
A TimeVector created with this constructor reassembles when the time is changed.
- Parameters
-
spc Space tlf Linear form to be evaluated when the time is changed
Definition at line 60 of file vectors.hh.
◆ TimeVector() [2/4]
|
inline |
Constructor.
A TimeVector created with this constructor does not reassemble when the time is changed.
- Parameters
-
spc Space
Definition at line 67 of file vectors.hh.
◆ TimeVector() [3/4]
|
inline |
Copy Constructor.
A TimeVector created with this constructor does not reassemble when the time is changed.
- Parameters
-
vec The Vector to be copied
Definition at line 74 of file vectors.hh.
◆ TimeVector() [4/4]
timestepping::TimeVector::TimeVector | ( | const concepts::Vector< Real > & | vec, |
const concepts::Formula< Real > & | frm, | ||
const Real | time = 0 |
||
) |
Constructor for a constant vector times a formula in time.
- Parameters
-
vec The vector frm The formula time Current time
◆ ~TimeVector()
|
inline |
Definition at line 85 of file vectors.hh.
Member Function Documentation
◆ add() [1/3]
|
virtualinherited |
◆ add() [2/3]
|
inlineinherited |
◆ add() [3/3]
|
inherited |
◆ apply()
|
inherited |
Application operator to each component, e.g.
std::sin or std::conj
◆ assemble()
|
inherited |
Assembles the vector w.r.t. linear form lf
and space spc
.
◆ data()
|
inlineinherited |
- Examples
- parallelizationTutorial.cc.
◆ fillEntries_()
|
protectedinherited |
◆ info()
|
protectedvirtualinherited |
◆ l1()
|
inherited |
l1 norm
◆ l2()
|
inlineinherited |
◆ l2_2()
|
inherited |
l2 norm squared
◆ max()
|
inherited |
Maximum of the absolute values in the vector, ie. norm.
◆ n()
|
inlineinherited |
◆ operator Real *()
|
inlineinherited |
◆ operator()() [1/2]
|
inlinevirtualinherited |
◆ operator()() [2/2]
|
inlinevirtualinherited |
◆ operator*() [1/2]
|
inherited |
Inner product (v
= this
, w
= \fnc)
or
for complex vectors respectivly.
◆ operator*() [2/2]
|
inherited |
◆ operator*=()
|
virtualinherited |
Scaling operator.
◆ operator+() [1/2]
|
inherited |
◆ operator+() [2/2]
|
inherited |
◆ operator+=() [1/2]
|
virtualinherited |
Addition operator.
◆ operator+=() [2/2]
|
virtualinherited |
◆ operator-() [1/2]
|
inherited |
◆ operator-() [2/2]
|
inherited |
◆ operator-=() [1/2]
|
virtualinherited |
Subtraction operator.
◆ operator-=() [2/2]
|
virtualinherited |
◆ operator/()
|
inherited |
◆ operator/=()
|
virtualinherited |
◆ resize()
|
inlinevirtualinherited |
◆ reverse()
|
inherited |
◆ set_()
|
private |
Sets the vector to vec
.
In difference to the constructor the rest remains.
◆ size()
|
inlineinherited |
- Examples
- parallelizationTutorial.cc.
◆ storeMatlab()
|
inherited |
Stores the vector in a Matlab sparse matrix.
◆ time() [1/2]
|
inline |
Returns the current time_
.
Definition at line 88 of file vectors.hh.
◆ time() [2/2]
void timestepping::TimeVector::time | ( | Real | time | ) |
Sets the current time_
.
If tlf_
is set, the entries are updated.
◆ updateEntries_()
|
inlineprivate |
Evaluates tlf_
at time_
.
Definition at line 106 of file vectors.hh.
◆ write()
|
inherited |
Writes the vector to a file.
This should be endian proof.
- Parameters
-
fname Filename
Member Data Documentation
◆ data_
|
protectedinherited |
◆ frm_
|
private |
Formula in time to multiply with template vector.
Definition at line 101 of file vectors.hh.
◆ spc_
|
private |
Space.
Definition at line 95 of file vectors.hh.
◆ storeMatlabCounter_
|
staticprivateinherited |
◆ template_
|
private |
Template vector.
Definition at line 103 of file vectors.hh.
◆ time_
|
private |
Time, is not necessarily correlated to the values of the vector.
Definition at line 99 of file vectors.hh.
◆ tlf_
|
private |
Linear form.
Definition at line 97 of file vectors.hh.
◆ v_
|
privateinherited |
The documentation for this class was generated from the following file:
- timestepping/vectors.hh