hp2D::TraceSpace Class Referenceabstract
Builds the trace space of an FE space. More...
#include <traces.hh>
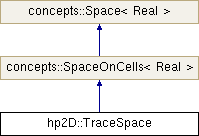
Public Types | |
typedef concepts::Scan< hp1D::BaseElement< Real > > | Scan |
typedef Scan< ElementWithCell< Real > > | Scanner |
enum | traceTypes { FIRST, MEAN, JUMP } |
typedef ElementWithCell< Real > | type |
typedef concepts::ElementAndFacette< hp2D::Element< Real > > | UnderlyingElement |
Public Member Functions | |
virtual uint | dim () const |
virtual uint | dim () const=0 |
Returns the dimension of the space. More... | |
virtual uint | getOutputDimension () const |
Returns the default output dimension, when we consider plotting a real-valued operator on this space. More... | |
virtual uint | nelm () const |
Returns the number of elements in the space. More... | |
virtual uint | nelm () const=0 |
Returns the number of elements in the space. More... | |
virtual void | recomputeShapefunctions () |
Recompute shape functions, e.g. More... | |
virtual Scan * | scan () const |
Returns a scanner to iterate over the elements of the space. More... | |
virtual Scanner * | scan () const=0 |
Returns a scanner to iterate over the elements of the space. More... | |
TraceSpace (concepts::SpaceOnCells< Real > &spc, const concepts::CellConditions *cc=0, enum traceTypes type=FIRST, const concepts::EdgeNormalVectorRule normalVectorRule=concepts::EdgeNormalVectorRule()) | |
Constructor. More... | |
TraceSpace (const concepts::SpaceOnCells< Real > &spc, const concepts::CellConditions *cc=0, enum traceTypes type=FIRST, const concepts::EdgeNormalVectorRule normalVectorRule=concepts::EdgeNormalVectorRule()) | |
TraceSpace (const concepts::SpaceOnCells< Real > &spc, const concepts::Set< uint > edgeAttr, enum traceTypes type=FIRST, const concepts::EdgeNormalVectorRule &normalVectorRule=concepts::EdgeNormalVectorRule()) | |
Constructor. More... | |
const concepts::HashMap< concepts::Sequence< UnderlyingElement > > | uelm () const |
Returns the mapping from the key of (topological) edge to the underlying 2D elements. More... | |
const concepts::Sequence< UnderlyingElement > | uelm (const concepts::Edge edge) const |
Returns the underlying 2D elements. More... | |
virtual | ~TraceSpace () |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Private Member Functions | |
template<class F > | |
bool | build_ (const Quad< Real > *elm, F condition) |
Builds elements for the edges w.r.t. More... | |
void | constructType_ (enum traceTypes type, const concepts::EdgeNormalVectorRule &normalVectorRule) |
template<class F > | |
void | test_ (const concepts::ElementWithCell< Real > &elm, F condition) |
Test if not implemented elements have edges w.r.t. More... | |
Private Attributes | |
const uint | dim_ |
Dimension of the FE space. More... | |
concepts::HashMap< hp1D::Element< Real > * > | edges_ |
Map from key of (topological) edge to the element. More... | |
concepts::Joiner< hp1D::BaseElement< Real > *, 1 > * | elm_ |
Linked list of the elements. More... | |
uint | nelm_ |
Number of elements currently active in the mesh. More... | |
std::unique_ptr< QuadEdgeBase > | quadEdge_ |
concepts::HashMap< concepts::Sequence< UnderlyingElement > > | uelm_ |
Map from key of (topological) edge to the underlying 2D elements. More... | |
bool | warn_edgeBuildMissElem_ |
Detailed Description
Builds the trace space of an FE space.
The elements are from type hp2D::Edge.
One can restrict the trace operation by cell conditions.
If the FE space is rebuild, one has to construct the trace space again.
Additonally a map from the topological edge to the set of the underlying elements is stored.
Member Typedef Documentation
◆ Scan
typedef concepts::Scan<hp1D::BaseElement<Real> > hp2D::TraceSpace::Scan |
◆ Scanner
|
inherited |
◆ type
|
inherited |
◆ UnderlyingElement
Member Enumeration Documentation
◆ traceTypes
Constructor & Destructor Documentation
◆ TraceSpace() [1/3]
hp2D::TraceSpace::TraceSpace | ( | const concepts::SpaceOnCells< Real > & | spc, |
const concepts::Set< uint > | edgeAttr, | ||
enum traceTypes | type = FIRST , |
||
const concepts::EdgeNormalVectorRule & | normalVectorRule = concepts::EdgeNormalVectorRule() |
||
) |
Constructor.
- Parameters
-
spc underlying space edgeAttr set of edge attributes
◆ TraceSpace() [2/3]
hp2D::TraceSpace::TraceSpace | ( | concepts::SpaceOnCells< Real > & | spc, |
const concepts::CellConditions * | cc = 0 , |
||
enum traceTypes | type = FIRST , |
||
const concepts::EdgeNormalVectorRule | normalVectorRule = concepts::EdgeNormalVectorRule() |
||
) |
Constructor.
- Parameters
-
spc underlying space cc build trace space w.r.t. cell conditions
If no cell conditions are given, the trace to all edges in the mesh is taken.
◆ TraceSpace() [3/3]
hp2D::TraceSpace::TraceSpace | ( | const concepts::SpaceOnCells< Real > & | spc, |
const concepts::CellConditions * | cc = 0 , |
||
enum traceTypes | type = FIRST , |
||
const concepts::EdgeNormalVectorRule | normalVectorRule = concepts::EdgeNormalVectorRule() |
||
) |
◆ ~TraceSpace()
|
virtual |
Member Function Documentation
◆ build_()
|
private |
Builds elements for the edges w.r.t.
to condition
of the element elm
, if it is a hp2D::Quad.
◆ constructType_()
|
private |
◆ dim() [1/2]
◆ dim() [2/2]
|
pure virtualinherited |
Returns the dimension of the space.
Implements concepts::Space< Real >.
Implemented in hp2D::hpAdaptiveSpace< Real >, hp3D::Space, hp2Dedge::Space, hp2D::Space, hp2D::hpAdaptiveSpace< Real >, and hp1D::Space.
◆ getOutputDimension()
|
inlinevirtualinherited |
◆ info()
|
protectedvirtual |
Reimplemented from concepts::SpaceOnCells< Real >.
◆ nelm() [1/2]
|
inlinevirtual |
◆ nelm() [2/2]
|
pure virtualinherited |
Returns the number of elements in the space.
Implements concepts::Space< Real >.
Implemented in hp2D::hpAdaptiveSpace< Real >, hp3D::Space, hp2Dedge::Space, hp2D::Space, hp2D::hpAdaptiveSpace< Real >, and hp1D::Space.
◆ recomputeShapefunctions()
|
virtual |
Recompute shape functions, e.g.
for other abscissas redefined through setIntegrationRule
◆ scan() [1/2]
|
inlinevirtual |
Returns a scanner to iterate over the elements of the space.
- Examples
- inhomDirichletBCsLagrange.cc.
◆ scan() [2/2]
|
pure virtualinherited |
Returns a scanner to iterate over the elements of the space.
Implements concepts::Space< Real >.
Implemented in hp2D::hpAdaptiveSpace< Real >, hp3D::Space, hp2Dedge::Space, hp2D::Space, hp2D::hpAdaptiveSpace< Real >, and hp1D::Space.
◆ test_()
|
private |
Test if not implemented elements have edges w.r.t.
to condition
and throws an exception in that case.
◆ uelm() [1/2]
|
inline |
◆ uelm() [2/2]
|
inline |
Member Data Documentation
◆ dim_
|
private |
◆ edges_
|
private |
◆ elm_
|
private |
◆ nelm_
|
private |
◆ quadEdge_
|
private |
◆ uelm_
|
private |
◆ warn_edgeBuildMissElem_
The documentation for this class was generated from the following file:
- hp2D/traces.hh