concepts::Triangle2d Class Reference
A 2D cell: triangle. More...
#include <cell2D.hh>
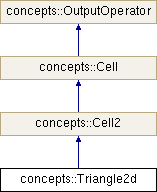
Classes | |
struct | Index |
Subclass of Triangle2d representing its index. More... | |
Public Types | |
typedef uint | index_type |
Public Member Functions | |
Real | area () const |
Returns the area of the element. More... | |
Real3d | center () const |
Returns the center of the cell. More... | |
Real2d | chi (Real xi, Real eta) const |
Evaluates the element map. More... | |
virtual Triangle2d * | child (uint i) |
Returns a child. More... | |
virtual const Triangle2d * | child (uint i) const |
Returns a child. More... | |
Triangle2d * | clone (Triangle &cntr, MappingTriangle2d *map) const |
Returns a copy of itself. More... | |
Triangle & | connector () const |
Returns the triangle connector (topology) More... | |
virtual Real3d | elemMap (const Real coord_local) const |
Element map from point local coordinates in 1D. More... | |
Real3d | elemMap (const Real2d &coord_local) const |
Element map from point local coordinates in 2D. More... | |
virtual Real3d | elemMap (const Real3d &coord_local) const |
Element map from point local coordinates in 3D. More... | |
bool | hasChildren () const |
Returns true if there is a least one child. More... | |
MapReal2d | jacobian (Real x, Real y) const |
Returns the Jacobian in a 2D linear map. More... | |
Real | jacobianDeterminant (const Real x, const Real y) const |
Returns determinant of the Jacobian. More... | |
MapReal2d | jacobianInverse (const Real x, const Real y) const |
Returns the inverse of the Jacobian in a 2D linear map. More... | |
ushort | level () const |
Returns the level of the cell. More... | |
const MappingTriangle2d * | map () const |
Returns the element map. More... | |
Triangle2d (Triangle &cntr, const MappingTriangle2d &map) | |
Constructor. More... | |
Real3d | vertex (uint i) const |
Returns the coordinates of the ith vertex. More... | |
virtual | ~Triangle2d () |
Static Public Member Functions | |
static Real2d | duffyInv (const Real2d &x) |
Maps coordinates from ![]() ![]() chi . More... | |
Static Public Attributes | |
static uint | MAX_LEVEL |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
Private Member Functions | |
Triangle2d (Triangle &cntr, MappingTriangle2d *map, const Index &idx) | |
Private constructor. More... | |
Private Attributes | |
Triangle2d * | chld_ |
Pointer to the first child. More... | |
Triangle & | cntr_ |
Reference to the triangle connector (topology) More... | |
Index | idx_ |
Index of this element. More... | |
Triangle2d * | lnk_ |
Pointer to a sibling. More... | |
MappingTriangle2d * | map_ |
Pointer to the element map. More... | |
Friends | |
std::ostream & | operator<< (std::ostream &os, const Triangle2d::Index &i) |
Detailed Description
A 2D cell: triangle.
If a triangle is subdivided, four new children are created.
- See also
- Cell for more information on cells in a mesh.
- Examples
- meshes.cc.
Member Typedef Documentation
◆ index_type
typedef uint concepts::Triangle2d::index_type |
Constructor & Destructor Documentation
◆ Triangle2d() [1/2]
concepts::Triangle2d::Triangle2d | ( | Triangle & | cntr, |
const MappingTriangle2d & | map | ||
) |
Constructor.
Takes the connector cntr
and the element map map
and creates a cell.
- Parameters
-
cntr Topological information of the triangle map Element map of the triangle
◆ ~Triangle2d()
|
virtual |
◆ Triangle2d() [2/2]
|
private |
Private constructor.
Member Function Documentation
◆ area()
Real concepts::Triangle2d::area | ( | ) | const |
Returns the area of the element.
◆ center()
|
inlinevirtual |
Returns the center of the cell.
Implements concepts::Cell2.
◆ chi()
Evaluates the element map.
Maps a corner from the unit square [0,1]2 onto the element. The three vertices are (0, 0), (1, 0) and (1, 1). If you already have coordinates in the triangle, use duffyInv
.
- Returns
- Point in 2D in physical coordinates.
- Parameters
-
xi eta
◆ child() [1/2]
|
virtual |
Returns a child.
If no children exist, four new children are created. The triangle is cut into four new triangles by joining the midpoints of its edges.
- Parameters
-
i Index of the child to be returned.
Implements concepts::Cell2.
◆ child() [2/2]
|
virtual |
Returns a child.
If no children exist, none are created and 0 is returned.
- Parameters
-
i Index of the child to be returned.
Implements concepts::Cell2.
◆ clone()
|
inline |
◆ connector()
|
inlinevirtual |
Returns the triangle connector (topology)
Implements concepts::Cell2.
◆ duffyInv()
Maps coordinates from to
to use them in
chi
.
◆ elemMap() [1/3]
Element map from point local coordinates in 1D.
Reimplemented in concepts::Edge2d, concepts::Edge1d, concepts::Sphere3d, and concepts::SphericalSurface3d.
◆ elemMap() [2/3]
Element map from point local coordinates in 2D.
Implements concepts::Cell2.
◆ elemMap() [3/3]
Element map from point local coordinates in 3D.
Reference element is 2D, third component is omitted.
Reimplemented from concepts::Cell.
◆ hasChildren()
|
inlineinherited |
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Implements concepts::Cell.
◆ jacobian()
Returns the Jacobian in a 2D linear map.
The input is a point from [0,1]2, the output is the Jacobian of the map at the given point. The Jacobian includes the map from into the physical coordinates but not the map from
to
.
- Parameters
-
x y
◆ jacobianDeterminant()
Returns determinant of the Jacobian.
- See also
- jacobian
◆ jacobianInverse()
Returns the inverse of the Jacobian in a 2D linear map.
- See also
- jacobian
◆ level()
|
inline |
◆ map()
|
inline |
◆ vertex()
|
virtual |
Returns the coordinates of the ith vertex.
Implements concepts::Cell2.
Friends And Related Function Documentation
◆ operator<<
|
friend |
Member Data Documentation
◆ chld_
|
private |
◆ cntr_
|
private |
◆ idx_
|
private |
◆ lnk_
|
private |
◆ map_
|
private |
◆ MAX_LEVEL
The documentation for this class was generated from the following file:
- geometry/cell2D.hh