concepts::FormulaPMLHamburger Class Referenceabstract
Class providing the formulas for hamburger PML. More...
#include <pml_formula.hh>
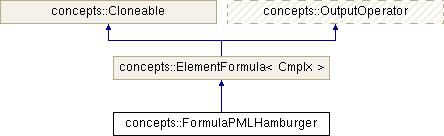
Public Types | |
enum | PMLMode { M1, M2, M3, M4, IDENT } |
typedef Cmplx | value_type |
Public Member Functions | |
virtual FormulaPMLHamburger * | clone () const |
Virtual constructor. More... | |
virtual ElementFormula< Cmplx, typename Realtype< Cmplx >::type > * | clone () const=0 |
Virtual copy constructor. More... | |
FormulaPMLHamburger (const Real R_, const Real d_, const int power_, const Real sigma0_, const Real center_x_, const Real center_y_, PMLMode mode_) | |
virtual Cmplx | operator() (const ElementWithCell< Real > &elm, const Real p, const Real t=0.0) const |
virtual Cmplx | operator() (const ElementWithCell< Real > &elm, const Real2d &p, const Real t=0.0) const |
virtual Cmplx | operator() (const ElementWithCell< Real > &elm, const Real3d &p, const Real t=0.0) const |
template<class RealNd > | |
Cmplx | operator() (const ElementWithCell< Real > &elm, const RealNd &p, Real2d px, const Real t=0.0) const |
virtual Cmplx | operator() (const ElementWithCell< typename Realtype< Cmplx >::type > &elm, const Real p, const Real t=0.0) const=0 |
Evaluates the formula. More... | |
virtual Cmplx | operator() (const ElementWithCell< typename Realtype< Cmplx >::type > &elm, const Real2d &p, const Real t=0.0) const=0 |
Evaluates the formula. More... | |
virtual Cmplx | operator() (const ElementWithCell< typename Realtype< Cmplx >::type > &elm, const Real3d &p, const Real t=0.0) const=0 |
Evaluates the formula. More... | |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
Private Member Functions | |
FormulaPMLCartNew::PMLMode | convert_mode_to_cart (FormulaPMLHamburger::PMLMode mode) |
FormulaPMLRadia::PMLMode | convert_mode_to_radia (FormulaPMLHamburger::PMLMode mode) |
Private Attributes | |
FormulaPMLCartNew | Cart |
Real2d | center_point_down |
Real2d | center_point_up |
Real | center_x |
Real | center_y |
Real | d |
PMLMode | mode |
int | power |
Real | R |
FormulaPMLRadia | RadiaDown |
FormulaPMLRadia | RadiaUp |
Real | sigma0 |
Detailed Description
Class providing the formulas for hamburger PML.
Definition at line 687 of file pml_formula.hh.
Member Typedef Documentation
◆ value_type
|
inherited |
Definition at line 37 of file elementFormula.hh.
Member Enumeration Documentation
◆ PMLMode
Enumerator | |
---|---|
M1 | |
M2 | |
M3 | |
M4 | |
IDENT |
Definition at line 689 of file pml_formula.hh.
Constructor & Destructor Documentation
◆ FormulaPMLHamburger()
|
inline |
Definition at line 727 of file pml_formula.hh.
Member Function Documentation
◆ clone() [1/2]
|
inlinevirtual |
Virtual constructor.
Returns a pointer to a copy of itself. The caller is responsible to destroy this copy.
Implements concepts::Cloneable.
Definition at line 762 of file pml_formula.hh.
◆ clone() [2/2]
|
pure virtualinherited |
Virtual copy constructor.
◆ convert_mode_to_cart()
|
inlineprivate |
Definition at line 702 of file pml_formula.hh.
◆ convert_mode_to_radia()
|
inlineprivate |
Definition at line 714 of file pml_formula.hh.
◆ info()
|
inlineprotectedvirtual |
Returns information in an output stream.
Reimplemented from concepts::OutputOperator.
Definition at line 806 of file pml_formula.hh.
◆ operator()() [1/7]
|
inlinevirtual |
Definition at line 778 of file pml_formula.hh.
◆ operator()() [2/7]
|
inlinevirtual |
Definition at line 772 of file pml_formula.hh.
◆ operator()() [3/7]
|
inlinevirtual |
Definition at line 766 of file pml_formula.hh.
◆ operator()() [4/7]
|
inline |
Definition at line 786 of file pml_formula.hh.
◆ operator()() [5/7]
|
pure virtualinherited |
◆ operator()() [6/7]
|
pure virtualinherited |
◆ operator()() [7/7]
|
pure virtualinherited |
Member Data Documentation
◆ Cart
|
private |
Definition at line 698 of file pml_formula.hh.
◆ center_point_down
|
private |
Definition at line 696 of file pml_formula.hh.
◆ center_point_up
|
private |
Definition at line 696 of file pml_formula.hh.
◆ center_x
|
private |
Definition at line 695 of file pml_formula.hh.
◆ center_y
|
private |
Definition at line 695 of file pml_formula.hh.
◆ d
|
private |
Definition at line 692 of file pml_formula.hh.
◆ mode
|
private |
Definition at line 697 of file pml_formula.hh.
◆ power
|
private |
Definition at line 693 of file pml_formula.hh.
◆ R
|
private |
Definition at line 691 of file pml_formula.hh.
◆ RadiaDown
|
private |
Definition at line 700 of file pml_formula.hh.
◆ RadiaUp
|
private |
Definition at line 699 of file pml_formula.hh.
◆ sigma0
|
private |
Definition at line 694 of file pml_formula.hh.
The documentation for this class was generated from the following file:
- waveprop/pml_formula.hh