concepts::BlendingHexahedron3d Class Reference
A 3D hexahedral element map for interpolation between arbitrary curved boundary quadrilateral elements according to the Linear Blending Function Method (Gordon and Hall, 1971). More...
#include <elementMaps3D.hh>
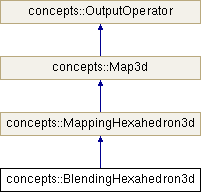
Public Member Functions | |
BlendingHexahedron3d (const BlendingHexahedron3d &v) | |
BlendingHexahedron3d (const MappingQuad3d *quadmap0, const MappingQuad3d *quadmap1, const MappingQuad3d *quadmap2, const MappingQuad3d *quadmap3, const MappingQuad3d *quadmap4, const MappingQuad3d *quadmap5, const Hexahedron &hex) | |
BlendingHexahedron3d (const Sequence< const MappingQuad3d * > quadmap, const Hexahedron &hex) | |
Constructor. More... | |
BlendingHexahedron3d * | clone () const override |
Returns a copy of the map. More... | |
MappingEdge3d * | edge (const uint edge) const |
MappingQuad3d * | face (const uint face) const override |
Returns the mapping of the given face. More... | |
MapReal3d | hessian (uint i, const Real x, const Real y, const Real z) const override |
Returns the Hessian, the integer indicates which 3x3 submap of the 3x3xi (i=1..3) tensor is required. More... | |
MapReal3d | jacobian (const Real x, const Real y, const Real z) const override |
Returns the jacobian of the element map. More... | |
virtual Real | jacobianDeterminant (const Real x, const Real y, const Real z) const |
Returns the determinant of the Jacobian. More... | |
virtual MapReal3d | jacobianInverse (const Real x, const Real y, const Real z) const |
Computes the inverse of the jacobian: More... | |
Real3d | operator() (Real x, Real y, Real z) const override |
Returns a point in 3D mapped from the unit cube [0,1]3 onto the element in physical 3d space. More... | |
MappingHexahedron3d * | part (const Real3d xi0, const Real3d xi1) const override |
~BlendingHexahedron3d () override | |
Protected Member Functions | |
std::ostream & | info (std::ostream &os) const override |
Returns information in an output stream. More... | |
Private Member Functions | |
void | construct_ (const std::array< const MappingQuad3d *, 6 > &quadmap, const Hexahedron &hex) |
Private Attributes | |
std::array< std::unique_ptr< MappingEdge3d >, 12 > | edgemap_ |
std::array< std::unique_ptr< MappingQuad3d >, 6 > | quadmap_ |
std::array< Real3d, 8 > | vtx_ |
Detailed Description
A 3D hexahedral element map for interpolation between arbitrary curved boundary quadrilateral elements according to the Linear Blending Function Method (Gordon and Hall, 1971).
Element parametrization:
where denotes a boolean operator sum of coordinate interpolation operators, e.g.
- See also
- BlendingQuad3d for a detailed explanation
- Todo:
move invertOrientation documentation to MappingQuad3d
homogenize construction with MappingQuad3d-iterators template <typename MappingQuad3dIterator> BlendingHexahedron3d( MappingQuad3dIterator begin, MappingQuad3dIterator end, const Hexahedron& hex);
Definition at line 242 of file elementMaps3D.hh.
Constructor & Destructor Documentation
◆ BlendingHexahedron3d() [1/3]
concepts::BlendingHexahedron3d::BlendingHexahedron3d | ( | const Sequence< const MappingQuad3d * > | quadmap, |
const Hexahedron & | hex | ||
) |
Constructor.
Every element of a given sequence of boundary quadrilateral mappings is cloned and reoriented from the quadrilateral orientations in the topological hexahedron to those induced by the coordinate directions in the hexahedral reference domain. IMPORTANT: The orientation of the quadrilateral element mappings in the input sequence must be aligned with the corresponding topological quadrilaterals. That is: The topological complex must be consistent with the geometric complex.
- Parameters
-
quadmap boundary element mappings forming the images of hex topological object corresponding to the element mapping to be constructed. Orientations in topological cell complex must be consistent with passed boundary element mappings in quadmap
◆ BlendingHexahedron3d() [2/3]
concepts::BlendingHexahedron3d::BlendingHexahedron3d | ( | const MappingQuad3d * | quadmap0, |
const MappingQuad3d * | quadmap1, | ||
const MappingQuad3d * | quadmap2, | ||
const MappingQuad3d * | quadmap3, | ||
const MappingQuad3d * | quadmap4, | ||
const MappingQuad3d * | quadmap5, | ||
const Hexahedron & | hex | ||
) |
◆ BlendingHexahedron3d() [3/3]
concepts::BlendingHexahedron3d::BlendingHexahedron3d | ( | const BlendingHexahedron3d & | v | ) |
◆ ~BlendingHexahedron3d()
|
override |
Member Function Documentation
◆ clone()
|
inlineoverridevirtual |
Returns a copy of the map.
Implements concepts::MappingHexahedron3d.
Definition at line 276 of file elementMaps3D.hh.
◆ construct_()
|
private |
◆ edge()
MappingEdge3d* concepts::BlendingHexahedron3d::edge | ( | const uint | edge | ) | const |
◆ face()
|
overridevirtual |
Returns the mapping of the given face.
Implements concepts::MappingHexahedron3d.
◆ hessian()
|
overridevirtual |
Returns the Hessian, the integer indicates which 3x3 submap of the 3x3xi (i=1..3) tensor is required.
Implements concepts::MappingHexahedron3d.
◆ info()
|
overrideprotectedvirtual |
Returns information in an output stream.
Implements concepts::MappingHexahedron3d.
◆ jacobian()
◆ jacobianDeterminant()
|
inlinevirtualinherited |
Returns the determinant of the Jacobian.
Reimplemented in concepts::MapHexahedron3d.
Definition at line 200 of file elementMaps3D.hh.
◆ jacobianInverse()
|
inlinevirtualinherited |
Computes the inverse of the jacobian:
Reimplemented in concepts::MapHexahedron3d.
Definition at line 196 of file elementMaps3D.hh.
◆ operator()()
Returns a point in 3D mapped from the unit cube [0,1]3 onto the element in physical 3d space.
- Returns
Implements concepts::MappingHexahedron3d.
◆ part()
|
overridevirtual |
- Todo:
- part supposed to be implemented for parent class
Implements concepts::MappingHexahedron3d.
Member Data Documentation
◆ edgemap_
|
private |
Definition at line 291 of file elementMaps3D.hh.
◆ quadmap_
|
private |
Definition at line 289 of file elementMaps3D.hh.
◆ vtx_
|
private |
Definition at line 293 of file elementMaps3D.hh.
The documentation for this class was generated from the following file:
- geometry/elementMaps3D.hh