hp3D::TraceSpace Class Referenceabstract
Builds the trace space of a FE space consisting of hexahedral elements. More...
#include <traces.hh>
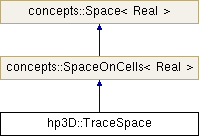
Public Types | |
typedef concepts::Scan< hp2D::Element< Real > > | Scan |
typedef Scan< ElementWithCell< Real > > | Scanner |
enum | traceTypes { FIRST, MEAN, JUMP } |
typedef ElementWithCell< Real > | type |
typedef concepts::ElementAndFacette< hp3D::Element< Real > > | UnderlyingElement |
Public Member Functions | |
virtual uint | dim () const |
virtual uint | dim () const=0 |
Returns the dimension of the space. More... | |
concepts::Set< uint > | getDofIds () const |
Returns the DOF indices belonging to the trace space. More... | |
virtual uint | getOutputDimension () const |
Returns the default output dimension, when we consider plotting a real-valued operator on this space. More... | |
virtual uint | nelm () const |
Returns the number of elements in the space. More... | |
virtual uint | nelm () const=0 |
Returns the number of elements in the space. More... | |
virtual void | recomputeShapeFunctions () |
virtual Scan * | scan () const |
Returns a scanner to iterate over the elements. More... | |
virtual Scanner * | scan () const=0 |
Returns a scanner to iterate over the elements of the space. More... | |
TraceSpace (const concepts::SpaceOnCells< Real > &spc, const concepts::Set< uint > faceAttr, enum traceTypes type=FIRST, const concepts::FaceNormalVectorRule &normalVectorRule=concepts::FaceNormalVectorRule()) | |
Constructor. More... | |
const concepts::HashMap< concepts::Sequence< UnderlyingElement > > | uelm () const |
Returns the mapping to the underlying 3D elements. More... | |
const concepts::Sequence< UnderlyingElement > | uelm (const concepts::Quad &quad) const |
Returns the underlying 3D elements. More... | |
virtual | ~TraceSpace () |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Private Member Functions | |
template<class F > | |
bool | build_ (const Hexahedron *elm, F condition) |
void | setType_ (enum traceTypes type, const concepts::FaceNormalVectorRule &normalVectorRule) |
Private Attributes | |
const uint | dim_ |
Dimension of the FE space. More... | |
concepts::Joiner< hp2D::Element< Real > *, 1 > * | elm_ |
Linked list of the elements. More... | |
concepts::HashMap< hp2D::Quad< Real > * > | faces_ |
Map from key of (topological) face to the element. More... | |
std::unique_ptr< HexahedronFaceBase > | faceTransfer_ |
Handler for the transfer of dofs of the Hexahedron to the dofs of the face. More... | |
uint | nelm_ |
Number of elements currently active in the space. More... | |
concepts::HashMap< concepts::Sequence< UnderlyingElement > > | uelm_ |
Map from key of (topological) face to the underlying element. More... | |
Detailed Description
Builds the trace space of a FE space consisting of hexahedral elements.
Member Typedef Documentation
◆ Scan
typedef concepts::Scan<hp2D::Element<Real> > hp3D::TraceSpace::Scan |
◆ Scanner
|
inherited |
◆ type
|
inherited |
◆ UnderlyingElement
Member Enumeration Documentation
◆ traceTypes
Constructor & Destructor Documentation
◆ TraceSpace()
hp3D::TraceSpace::TraceSpace | ( | const concepts::SpaceOnCells< Real > & | spc, |
const concepts::Set< uint > | faceAttr, | ||
enum traceTypes | type = FIRST , |
||
const concepts::FaceNormalVectorRule & | normalVectorRule = concepts::FaceNormalVectorRule() |
||
) |
Constructor.
- Parameters
-
spc The underlying space. faceAttr Set of face attibutes which define the trace space.
◆ ~TraceSpace()
|
virtual |
Member Function Documentation
◆ build_()
|
private |
◆ dim() [1/2]
◆ dim() [2/2]
|
pure virtualinherited |
Returns the dimension of the space.
Implements concepts::Space< Real >.
Implemented in hp2D::hpAdaptiveSpace< Real >, hp3D::Space, hp2Dedge::Space, hp2D::Space, hp2D::hpAdaptiveSpace< Real >, and hp1D::Space.
◆ getDofIds()
concepts::Set<uint> hp3D::TraceSpace::getDofIds | ( | ) | const |
Returns the DOF indices belonging to the trace space.
◆ getOutputDimension()
|
inlinevirtualinherited |
◆ info()
|
protectedvirtual |
Reimplemented from concepts::SpaceOnCells< Real >.
◆ nelm() [1/2]
|
inlinevirtual |
◆ nelm() [2/2]
|
pure virtualinherited |
Returns the number of elements in the space.
Implements concepts::Space< Real >.
Implemented in hp2D::hpAdaptiveSpace< Real >, hp3D::Space, hp2Dedge::Space, hp2D::Space, hp2D::hpAdaptiveSpace< Real >, and hp1D::Space.
◆ recomputeShapeFunctions()
|
virtual |
◆ scan() [1/2]
|
inlinevirtual |
◆ scan() [2/2]
|
pure virtualinherited |
Returns a scanner to iterate over the elements of the space.
Implements concepts::Space< Real >.
Implemented in hp2D::hpAdaptiveSpace< Real >, hp3D::Space, hp2Dedge::Space, hp2D::Space, hp2D::hpAdaptiveSpace< Real >, and hp1D::Space.
◆ setType_()
|
private |
◆ uelm() [1/2]
|
inline |
◆ uelm() [2/2]
|
inline |
Member Data Documentation
◆ dim_
|
private |
◆ elm_
|
private |
◆ faces_
|
private |
◆ faceTransfer_
|
private |
Handler for the transfer of dofs of the Hexahedron to the dofs of the face.
◆ nelm_
|
private |
◆ uelm_
|
private |
The documentation for this class was generated from the following file:
- hp3D/traces.hh