eigensolver::JdbSym Class Referenceabstract
Eigenvalue solver using JDBSYM. More...
#include <jdbsym.hh>
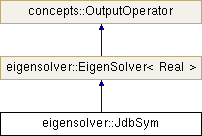
Public Member Functions | |
virtual uint | converged () const |
virtual uint | converged () const=0 |
Returns the number of converged eigen pairs. More... | |
virtual const concepts::Array< concepts::Vector< Real > * > & | getEF () |
virtual const concepts::Array< Real > & | getEV () |
Returns an array with the eigen values. More... | |
virtual uint | iterations () const |
virtual uint | iterations () const=0 |
Returns the number of iterations. More... | |
JdbSym (concepts::Operator< Real > &stiff, concepts::Operator< Real > &mass, Real tol, uint maxit=150, Real tau=0.0, uint jdtype=1, uint kmax=1, concepts::SolverFabric< Real > *fabric=0, const concepts::Array< concepts::Vector< Real > * > *start=0) | |
Constructor. More... | |
virtual | ~JdbSym () |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
Private Member Functions | |
void | compute_ () |
The real compute routine. More... | |
Private Attributes | |
bool | computed_ |
If false, the code will have to compute, otherwise not. More... | |
concepts::Array< Real > | eigenvectors_ |
Storage space for eigenvectors. More... | |
concepts::Array< concepts::Vector< Real > * > | ev_ |
References into storage for eigenvectors. More... | |
concepts::SolverFabric< Real > * | fabric_ |
int | it_ |
Actual number of iterations. More... | |
const uint | jdtype_ |
Type of eigen value solver. More... | |
int | k_conv_ |
Number of converged eigenpais. More... | |
uint | kmax_ |
Number of eigenvalues. More... | |
concepts::Array< Real > | lambda_ |
Storage space for eigenvalues. More... | |
concepts::Operator< Real > & | mass_ |
Mass matrix (ie. More... | |
uint | massCounter_ |
uint | maxit_ |
Maximal number of iterations. More... | |
const concepts::Array< concepts::Vector< Real > * > * | start_ |
concepts::Operator< Real > & | stiff_ |
Stiffness matrix (ie. discretazation of spatial problem) More... | |
uint | stiffCounter_ |
Statistics, counts number of evaluations of matrix vector product. More... | |
Real | tau_ |
Target value for the eigenvalues. More... | |
Real | tol_ |
Tolerance. More... | |
Static Private Attributes | |
static int | jdbsymLock_ |
Lock for the eigen value solver. More... | |
Detailed Description
Eigenvalue solver using JDBSYM.
JDBSYM is an implementation of the Jacobi-Davidson method optimized for symmetric eigenvalue problems. It solves eigenproblems of the form and
with or without preconditioning, where A is symmetric and B is symmetric positive definite. The implementation supports blocking.
- See also
- Roman Geus and Oscar Chinellato, JDBSYM 0.14, 2000.
- Roman Geus. The Jacobi-Davidson algorithm for solving large sparse symmetric eigenvalue problems with application to the design of accelerator cavities. PhD thesis 14734, Swiss Federal Institute of Technology Zurich, 2002.
Constructor & Destructor Documentation
◆ JdbSym()
eigensolver::JdbSym::JdbSym | ( | concepts::Operator< Real > & | stiff, |
concepts::Operator< Real > & | mass, | ||
Real | tol, | ||
uint | maxit = 150 , |
||
Real | tau = 0.0 , |
||
uint | jdtype = 1 , |
||
uint | kmax = 1 , |
||
concepts::SolverFabric< Real > * | fabric = 0 , |
||
const concepts::Array< concepts::Vector< Real > * > * | start = 0 |
||
) |
Constructor.
Initializes the JdbSym class. As parameter it takes the few most important variables that are needed for the Jacobi-Davidson algorithm. The rest of the parameters are given as default and work in most cases. The class then solves the problem where A, B are the stiffness and the mass matrices respectively.
The computation is only perfomed if getEV
or getEF
is called.
- Parameters
-
stiff Stiffness matrix A mass Mass matrix B fabric Solver fabric for a linear solver (preconditioner for the shifted stiffness matrix) tol Convergence tolerance for the eigenpairs. For a pair convergence is defined by
maxit Maximal number of iterations tau Target value of Jacobi-Davidson algorithm. The code will find the kmax
eigenvalues closest totau
.jdtype Type of solver required. An older solver and a newer solver are possible to use. kmax Number of eigenpairs to be computed start Starting vectors. Used to build the initial search subspace
◆ ~JdbSym()
|
virtual |
Member Function Documentation
◆ compute_()
|
private |
◆ converged() [1/2]
|
inlinevirtual |
◆ converged() [2/2]
|
pure virtualinherited |
Returns the number of converged eigen pairs.
Implemented in eigensolver::ArPack< Real >.
◆ getEF()
|
virtual |
Implements eigensolver::EigenSolver< Real >.
◆ getEV()
|
virtual |
Returns an array with the eigen values.
- Deprecated:
- : this interface requires that the returned array must be hold as a member variable of the class.
(use std::auto_pointer or similar)
Implements eigensolver::EigenSolver< Real >.
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Reimplemented from eigensolver::EigenSolver< Real >.
◆ iterations() [1/2]
|
inlinevirtual |
◆ iterations() [2/2]
|
pure virtualinherited |
Returns the number of iterations.
Implemented in eigensolver::ArPack< Real >.
Member Data Documentation
◆ computed_
|
private |
◆ eigenvectors_
|
private |
◆ ev_
|
private |
◆ fabric_
|
private |
◆ it_
|
private |
◆ jdbsymLock_
|
staticprivate |
◆ jdtype_
|
private |
◆ k_conv_
|
private |
◆ kmax_
|
private |
◆ lambda_
|
private |
◆ mass_
|
private |
◆ massCounter_
◆ maxit_
|
private |
◆ start_
|
private |
◆ stiff_
|
private |
◆ stiffCounter_
|
private |
◆ tau_
|
private |
◆ tol_
The documentation for this class was generated from the following file:
- eigensolver/jdbsym.hh