concepts::StiffArray< dim, F > Class Template Reference
An array of objects of fix length, defined by template parameter dim
.
More...
#include <stiffArray.hh>
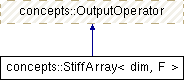
Public Member Functions | |
StiffArray< dim, F > & | apply (F &fnc(F &)) |
Application operator to each component, e.g. More... | |
Array< F > | array_data () const |
F * | data () |
Returns a pointer to the data in the array. More... | |
const F * | data () const |
Returns a pointer to the data in the array. More... | |
uint | length () |
operator Array< F > () const | |
operator const F * () const | |
Returns a pointer to the data in the array. More... | |
operator F* () | |
Returns a pointer to the data in the array. More... | |
StiffArray< dim, F > & | operator*= (const F n) |
Scaling operator. More... | |
StiffArray< dim, F > & | operator*= (const StiffArray< dim, F > &a) |
Multiplication operator. More... | |
StiffArray< dim, F > & | operator+= (const F n) |
Addition operator. More... | |
StiffArray< dim, F > & | operator-= (const F n) |
Subtraction operator. More... | |
StiffArray< dim, F > & | operator/= (const F n) |
Division operator. More... | |
StiffArray< dim, F > & | operator= (const F n) |
Assignement operator. More... | |
F & | operator[] (const int i) |
Index operator. More... | |
const F & | operator[] (const int i) const |
Index operator. More... | |
StiffArray () | |
Constructor. More... | |
StiffArray (const Array< F > &dft) | |
Constructor. More... | |
StiffArray (const F &dft) | |
Constructor. More... | |
StiffArray (const StiffArray< dim, F > &a) | |
Copy constructor. More... | |
template<class H > | |
StiffArray (const StiffArray< dim, H > &a, const F &fnc(const H &)) | |
Constructor. More... | |
template<class H > | |
StiffArray (const StiffArray< dim, H > &a, F fnc(const H &)) | |
Constructor. More... | |
void | zeros () |
Fills the memory with zeros. More... | |
~StiffArray () | |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
Private Attributes | |
F * | data_ |
Data. More... | |
Detailed Description
template<uint dim, class F>
class concepts::StiffArray< dim, F >
An array of objects of fix length, defined by template parameter dim
.
Is almost similar to Array, but without methods for resizing.
Definition at line 29 of file stiffArray.hh.
Constructor & Destructor Documentation
◆ StiffArray() [1/6]
|
inline |
Constructor.
Definition at line 32 of file stiffArray.hh.
◆ StiffArray() [2/6]
|
inline |
◆ StiffArray() [3/6]
|
inline |
◆ StiffArray() [4/6]
|
inline |
Constructor.
- Parameters
-
a Stiff array with elements of type H fnc Function which maps from H to F
Definition at line 52 of file stiffArray.hh.
◆ StiffArray() [5/6]
|
inline |
Constructor.
- Parameters
-
a Stiff array with elements of type H fnc Function which maps from H to F, e.g. std::real
Definition at line 63 of file stiffArray.hh.
◆ StiffArray() [6/6]
|
inline |
Copy constructor.
Definition at line 69 of file stiffArray.hh.
◆ ~StiffArray()
|
inline |
Definition at line 72 of file stiffArray.hh.
Member Function Documentation
◆ apply()
|
inline |
Application operator to each component, e.g.
std::sin or std::conj
Definition at line 140 of file stiffArray.hh.
◆ array_data()
|
inline |
Definition at line 92 of file stiffArray.hh.
◆ data() [1/2]
|
inline |
Returns a pointer to the data in the array.
Definition at line 85 of file stiffArray.hh.
◆ data() [2/2]
|
inline |
Returns a pointer to the data in the array.
Definition at line 87 of file stiffArray.hh.
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Reimplemented from concepts::OutputOperator.
Reimplemented in concepts::MultiIndex< dim >.
Definition at line 152 of file stiffArray.hh.
◆ length()
|
inline |
Definition at line 77 of file stiffArray.hh.
◆ operator Array< F >()
|
inline |
Definition at line 90 of file stiffArray.hh.
◆ operator const F *()
|
inline |
Returns a pointer to the data in the array.
Definition at line 83 of file stiffArray.hh.
◆ operator F*()
|
inline |
Returns a pointer to the data in the array.
Definition at line 81 of file stiffArray.hh.
◆ operator*=() [1/2]
|
inline |
Scaling operator.
Definition at line 108 of file stiffArray.hh.
◆ operator*=() [2/2]
|
inline |
Multiplication operator.
Definition at line 133 of file stiffArray.hh.
◆ operator+=()
|
inline |
Addition operator.
Definition at line 123 of file stiffArray.hh.
◆ operator-=()
|
inline |
Subtraction operator.
Definition at line 128 of file stiffArray.hh.
◆ operator/=()
|
inline |
Division operator.
Definition at line 113 of file stiffArray.hh.
◆ operator=()
|
inline |
Assignement operator.
Definition at line 118 of file stiffArray.hh.
◆ operator[]() [1/2]
|
inline |
Index operator.
Definition at line 101 of file stiffArray.hh.
◆ operator[]() [2/2]
|
inline |
Index operator.
Definition at line 95 of file stiffArray.hh.
◆ zeros()
|
inline |
Fills the memory with zeros.
Definition at line 79 of file stiffArray.hh.
Member Data Documentation
◆ data_
|
private |
Data.
Definition at line 148 of file stiffArray.hh.
The documentation for this class was generated from the following file:
- toolbox/stiffArray.hh